emojiΒΆ
Release v2.11.1. (Installation)
emoji supports Python 3.6+. The last version to support Python 2.7 and 3.5 was v2.4.0.
Usage and ExamplesΒΆ
The main purpose of this package is converting Unicode emoji to emoji names and vice
versa with emojize()
and demojize()
.
The entire set of Emoji codes as defined by the Unicode consortium
is supported in addition to a bunch of aliases.
By default, only the official list is enabled but doing emoji.emojize(language='alias')
enables both the full list and aliases.
>>> print(emoji.emojize('Python is :thumbs_up:'))
Python is π
>>> print(emoji.emojize('Python is :thumbsup:', language='alias'))
Python is π
>>> print(emoji.demojize('Python is π'))
Python is :thumbs_up:
>>> print(emoji.emojize("Python is fun :red_heart:", variant="text_type"))
Python is fun β€οΈ
>>> print(emoji.emojize("Python is fun :red_heart:", variant="emoji_type"))
Python is fun β€οΈ
LanguagesΒΆ
By default, the language is English (language='en'
) but also supported languages are:
Spanish ('es'
), Portuguese ('pt'
), Italian ('it'
), French ('fr'
), German ('de'
), Farsi/Persian ('fa'
)
>>> print(emoji.emojize('Python es :pulgar_hacia_arriba:', language='es'))
Python es π
>>> print(emoji.demojize('Python es π', language='es'))
Python es :pulgar_hacia_arriba:
>>> print(emoji.emojize("Python Γ© :polegar_para_cima:", language='pt'))
Python Γ© π
>>> print(emoji.demojize("Python Γ© π", language='pt'))
Python Γ© :polegar_para_cima:
Extracting emojiΒΆ
The function analyze()
finds all emoji in string and yields the emoji
together with its position and the available meta information about the emoji.
analyze()
returns a generator that yields each emoji, so you need to iterate or
convert the output to a list.
>>> first_token = next(emoji.analyze('Python is π'))
Token(chars='π', value=EmojiMatch(π, 10:11))
>>> emoji_match = first_token.value
EmojiMatch(π, 10:11)
>>> emoji_match.data
{'en': ':thumbs_up:', 'status': 2, 'E': 0.6, 'alias': [':thumbsup:', ':+1:'], 'variant': True, 'de': ':daumen_hoch:', 'es': ':pulgar_hacia_arriba:', 'fr': ':pouce_vers_le_haut:', 'ja': ':γ΅γ γΊγ’γγ:', 'ko': ':μ¬λ¦°_μμ§:', 'pt': ':polegar_para_cima:', 'it': ':pollice_in_su:', 'fa': ':ΩΎΨ³ΩΨ―ΫΨ―Ω:', 'id': ':jempol_ke_atas:', 'zh': ':ζζεδΈ:'}
>>> list(emoji.analyze('A π©βπ aboard a π'))
[Token(chars='π©\u200dπ', value=EmojiMatch(π©βπ, 2:5)), Token(chars='π', value=EmojiMatch(π, 15:16))]
>>> list(emoji.analyze('Aπ©βπBπ', non_emoji=True))
[Token(chars='A', value='A'), Token(chars='π©\u200dπ', value=EmojiMatch(π©βπ, 1:4)), Token(chars='B', value='B'), Token(chars='π', value=EmojiMatch(π, 5:6))]
The parameter join_emoji
controls whether non-RGI emoji are handled as a single token or as multiple emoji:
>>> list(emoji.analyze('π¨βπ©πΏβπ§π»βπ¦πΎ', join_emoji=True))
[Token(chars='π¨\u200dπ©πΏ\u200dπ§π»\u200dπ¦πΎ', value=EmojiMatchZWJNonRGI(π¨βπ©πΏβπ§π»βπ¦πΎ, 0:10))]
>>> list(emoji.analyze('π¨βπ©πΏβπ§π»βπ¦πΎ', join_emoji=False))
[Token(chars='π¨', value=EmojiMatch(π¨, 0:1)), Token(chars='π©πΏ', value=EmojiMatch(π©πΏ, 2:4)), Token(chars='π§π»', value=EmojiMatch(π§π», 5:7)), Token(chars='π¦πΎ', value=EmojiMatch(π¦πΎ, 8:10))]
The function emoji_list()
finds all emoji in string and their position.
Keep in mind that an emoji can span over multiple characters:
>>> emoji.emoji_list('Python is π')
[{'match_start': 10, 'match_end': 11, 'emoji': 'π'}]
>>> emoji.emoji_list('A π©βπ aboard a π')
[{'match_start': 2, 'match_end': 5, 'emoji': 'π©βπ'}, {'match_start': 15, 'match_end': 16, 'emoji': 'π'}]
To retrieve the distinct set of emoji from a string, use distinct_emoji_list()
:
>>> emoji.distinct_emoji_list('Some emoji: π, π, π, π, π, π¦οΈ')
['π', 'π', 'π¦οΈ', 'π']
To count the number of emoji in a string, use emoji_count()
:
>>> emoji.emoji_count('Some emoji: π, π, π, π, π, π¦οΈ')
6
>>> emoji.emoji_count('Some emoji: π, π, π, π, π, π¦οΈ', unique=True)
4
You can check if a string is a single, valid emoji with is_emoji()
>>> emoji.is_emoji('π')
True
>>> emoji.is_emoji('ππ')
False
>>> emoji.is_emoji('test')
False
While dealing with emojis, it is generally a bad idea to look at individual characters.
Unicode contains modifier characters, such as variation selectors, which are not emojis themselves
and modify the preceding emoji instead. You can check if a string has only emojis in it with purely_emoji()
>>> '\U0001f600\ufe0f'
'π'
>>> emoji.is_emoji('\U0001f600\ufe0f')
False
>>> emoji.is_emoji('\U0001f600')
True
>>> emoji.is_emoji('\ufe0f')
False
>>> emoji.purely_emoji('\U0001f600\ufe0f')
True
To get more information about an emoji, you can look it up in the EMOJI_DATA
dict:
pprint(emoji.EMOJI_DATA['π'])
{'E': 0.7,
'alias': [':earth_africa:'],
'de': ':globus_mit_europa_und_afrika:',
'en': ':globe_showing_Europe-Africa:',
'es': ':globo_terrΓ‘queo_mostrando_europa_y_Γ‘frica:',
'fr': ':globe_tournΓ©_sur_lβafrique_et_lβeurope:',
'it': ':europa_e_africa:',
'pt': ':globo_mostrando_europa_e_Γ‘frica:',
'status': 2,
'variant': True}
'E'
is the Emoji version.
'status'
is defined in STATUS
. For example 2
corresponds
to 'fully_qualified'
. More information on the meaning can be found in the
Unicode Standard http://www.unicode.org/reports/tr51/#Emoji_Variation_Selector_Notes
Replacing and removing emojiΒΆ
With replace_emoji()
you can replace, filter, escape or remove emoji in a string:
>>> emoji.replace_emoji('Python is π', replace='')
'Python is '
>>> emoji.replace_emoji('Python is π', replace='π')
'Python is π'
>>> def unicode_escape(chars, data_dict):
>>> return chars.encode('unicode-escape').decode()
>>> emoji.replace_emoji('Python is π', replace=unicode_escape)
'Python is \U0001f44d'
>>> def xml_escape(chars, data_dict):
>>> return chars.encode('ascii', 'xmlcharrefreplace').decode()
>>> emoji.replace_emoji('Python is π', replace=xml_escape)
'Python is 👍'
>>> emoji.replace_emoji('Python is π', replace=lambda chars, data_dict: chars.encode('ascii', 'namereplace').decode())
'Python is \N{THUMBS UP SIGN}'
>>> emoji.replace_emoji('Python is π', replace=lambda chars, data_dict: data_dict['es'])
'Python is :pulgar_hacia_arriba:'
Emoji versionsΒΆ
The parameter version
in replace_emoji()
allows to replace only emoji above
that Emoji version to prevent incompatibility with older platforms.
For the functions emojize()
and demojize()
the parameter version
will
replace emoji above the specified version with the value of the parameter handle_version
.
It defaults to an empty string, but can be set to any string or a function that returns a string.
For example the :croissant:
π₯ emoji was added in Emoji 3.0 (Unicode 9.0) in 2016 and
:T-Rex:
π¦ was added later in Emoji 5.0 (Unicode 10.0) in 2017:
>>> emoji.replace_emoji('A π¦ is eating a π₯', replace='[Unsupported emoji]', version=1.0)
'A [Unsupported emoji] is eating a [Unsupported emoji]'
>>> emoji.replace_emoji('A π¦ is eating a π₯', replace=lambda chars, data_dict: data_dict['en'], version=3.0)
'A :T-Rex: is eating a π₯'
>>> emoji.emojize('A :T-Rex: is eating a :croissant:', version=3.0)
'A is eating a π₯'
>>> emoji.emojize('A :T-Rex: is eating a :croissant:', version=3.0, handle_version='[Unsupported emoji]')
'A [Unsupported emoji] is eating a π₯'
>>> emoji.demojize('A π¦ is eating a π₯', version=3.0)
'A is eating a :croissant:'
>>> emoji.replace_emoji('A π¦ is eating a π₯', replace='', version=5.0)
'A π¦ is eating a π₯'
You can find the version of an emoji with version()
:
>>> emoji.version('π₯')
3
>>> emoji.version('ποΈββοΈ')
4
>>> emoji.version('π¦')
5
Non-RGI ZWJ emojiΒΆ
Some emoji contain multiple persons and each person can have an individual skin tone.
Unicode supports Multi-Person Skin Tones as of Emoji 11.0. Skin tones can be add to the nine characters known as Multi-Person Groupings.
Multi-person groups with different skin tones can be represented with Unicode, but are not yet RGI (recommended for general interchange). This means Unicode.org recommends not to show them in emoji keyboards. However some browser and platforms already support some of them:
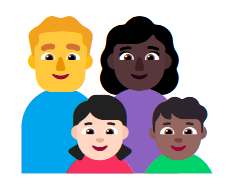
The emoji π¨βπ©πΏβπ§π»βπ¦πΎ as it appears in Firefox on Windows 11ΒΆ
It consists of eleven Unicode characters, four person emoji, four different skin tones joined together by three \u200d
Zero-Width Joiner:
π¨
:man:
π½
:medium_skin_tone:
\u200d
π©
:woman:
πΏ
:dark_skin_tone:
\u200d
π§
:girl:
π»
:light_skin_tone:
\u200d
π¦
:boy:
πΎ
:medium-dark_skin_tone:
On platforms that donβt support it, it might appear as separate emoji: π¨π½π©πΏπ§π»π¦πΎ
In the module configuration config
you can control how such emoji are handled.
Migrating to version 2.0.0ΒΆ
There a two major, breaking changes in version 2.0.0
non-English short codesΒΆ
The names of emoji in non-English languages have changed, because the data files were updated to the new version 41. See https://cldr.unicode.org/index/downloads.
That means some :short-code-emoji:
with non-English names will no longer work in 2.0.0.
emojize()
will ignore the old codes.
This may be a problem if you have previously stored :short-code-emoji:
with non-English names
for example in a database or if your users have stored them.
Regular expressionΒΆ
The function get_emoji_regexp()
was removed in 2.0.0. Internally the module no longer uses
a regular expression when scanning for emoji in a string (e.g. in demojize()
).
The regular expression was slow in Python 3 and it failed to correctly find certain combinations of long emoji (emoji consisting of multiple Unicode codepoints).
If you used the regular expression to remove emoji from strings, you can use replace_emoji()
as shown in the examples above.
If you want to extract emoji from strings, you can use emoji_list()
as a replacement.
If you want to keep using a regular expression despite its problems, you can create the expression yourself like this:
import re
import emoji
def get_emoji_regexp():
# Sort emoji by length to make sure multi-character emojis are
# matched first
emojis = sorted(emoji.EMOJI_DATA, key=len, reverse=True)
pattern = '(' + '|'.join(re.escape(u) for u in emojis) + ')'
return re.compile(pattern)
exp = get_emoji_regexp()
print(exp.sub(repl='[emoji]', string='A ποΈββοΈ is eating a π₯'))
Output:
A [emoji] is eating a [emoji]
Common problemsΒΆ
UnicodeWarning: Unicode unequal comparison failed to convert both arguments to Unicode - interpreting them as being unequal
This exception is thrown in Python 2.7 if you passed a str
string instead of a
unicode
string.
You should only pass Unicode strings to this module.
See https://python.readthedocs.io/en/v2.7.2/howto/unicode.html#the-unicode-type for more information on Unicode in Python 2.7.
The API documentationΒΆ
Reference documentation of all functions and properties in the module:
API Reference |
|
---|---|
Functions: |
|
Replace emoji names with Unicode codes |
|
Replace Unicode emoji with emoji shortcodes |
|
Find Unicode emoji in a string |
|
Replace Unicode emoji with a customizable string |
|
Location of all emoji in a string |
|
Distinct list of emojis in the string |
|
Number of emojis in a string |
|
Check if a string/character is a single emoji |
|
Check if a string contains only emojis |
|
Find Unicode/Emoji version of an emoji |
|
Module variables: |
|
Dict of all emoji |
|
Dict of Unicode/Emoji status |
|
Module wide configuration |
|
Classes: |
|
LinksΒΆ
Overview of all emoji:
https://carpedm20.github.io/emoji/
(auto-generated list of the emoji that are supported by the current version of this package)
For English:
For Spanish:
For Portuguese:
For Italian:
For French:
For German: